Suppose you have a number of pdf files and you want to combine them to create combinations of them according to some rules. An example is for teachers that have grouped exercices based on difficulty level and want to create automatically different tests
So, assume that you have 4 levels of difficulty: A, B, C, D and you have 6 exercises of Level A, and 4 for each of the other levels
So, if we define this in a programming language like python we will have this:
a = ["1", "2", "3", "4", "5", "6"],
["7", "8", "9", "10"],
["11", "12", "13", "14"],
["15", "16", "17","18"]
So we create some folders and put the files like this
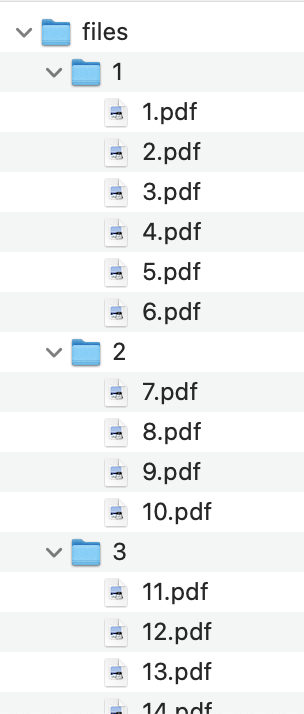
So, actually we need the cartesian product with all the combination of files. Then we have to read them one by one and browse files and create one pdf per combination
import itertools
from PyPDF2 import PdfFileMerger
from os import listdir
import os
input_dir = "somedirectory_for_input"
output_dir= "somedirectory_for_output"
separator = ''
a = ["1", "2", "3", "4", "5", "6"],["7", "8", "9", "10"],["11", "12", "13", "14"], ["15", "16", "17","18"]
b = list(itertools.product(*a))
for i in b:
#print(i[1] + " " + i[0])
path = output_dir + separator.join(i) + ".pdf"
merge_list=[]
merge_list.append(input_dir + '1/' + i[0] + ".pdf")
merge_list.append(input_dir + '2/' + i[1] + ".pdf")
merge_list.append(input_dir + '3/' + i[2] + ".pdf")
merge_list.append(input_dir + '4/' + i[3] + ".pdf")
merger = PdfFileMerger()
for pdf in merge_list:
merger.append(pdf)
if not os.path.exists(path):
merger.write(path)
merger.close()
Now we will have in our output folder one file per combination
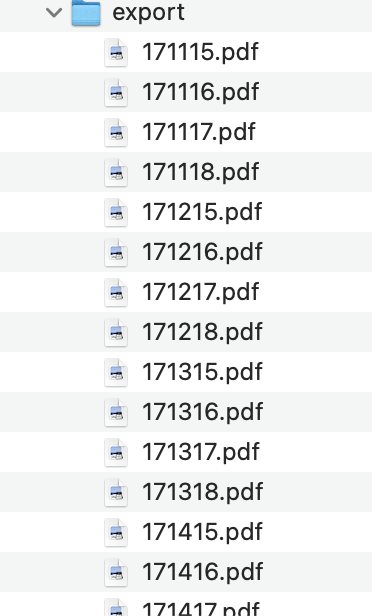